C2DArray Class¶
Overview¶
Note
The C2DArray class handles 2D (two dimensional) arrays for handling .2da
text files that store game data (typically rules etc) in columns and rows. See 2DA file format for details.
The structure used for this class is C2DArray
Quick Reference¶
Quick Ref |
void C2DArray::~C2DArray() |
unsigned char C2DArray::Find(CString& sSearchString, CString& sXLabel, CString& sYLabel, unsigned char bSearchLabels) |
unsigned char C2DArray::Find(CString& sSearchString, CPoint& ptLocation, unsigned char bSearchLabels) |
const long C2DArray::GetAtLong(CPoint& coordinates) |
void C2DArray::Load(CResRef& res) |
void C2DArray::LoadLines(CResRef& res) |
Constructors¶
Name |
Description |
Destroys a |
C2DArray::~C2DArray¶
Destroys the C2DArray
object.
~C2DArray();
Remarks
Destroys the C2DArray
object.
Methods¶
Name |
Description |
Locate a text value in the 2da array using column and row text headers to limit search |
|
Return a text value from the specified x and y coords of the 2da array |
|
Return a long integer value from the specified x and y coords of the 2da array |
|
Load a 2da resource into the C2DArray class |
|
Load a 2da resource into the C2DArray class |
C2DArray::Find¶
Locates a text value in the 2da array using column and row text headers to limit search.
unsigned char C2DArray::Find(
CString& sSearchString,
CString& sXLabel,
CString& sYLabel,
unsigned char bSearchLabels);
Parameters
CString& sSearchString - text string to search for
CString& sXLabel - column to search
CString& sYLabel - row to search
unsigned char
bSearchLabels - search among labels for sSearchString
Return Value
Returns the result of the search as an unsigned char.
Remarks
bSearchLabels can be set to TRUE
to search labels.
C2DArray::Find¶
Locate a text value in the 2da array using x and y coords to limit search
unsigned char C2DArray::Find(
CString& sSearchString,
CPoint& ptLocation,
unsigned char bSearchLabels);
Parameters
CString& sSearchString - text string to search for
CPoint& ptLocation - x and y coordinates to search
unsigned char
bSearchLabels - search among labels for sSearchString
Return Value
Returns the result of the search as an unsigned char.
Remarks
bSearchLabels can be set to TRUE
to search labels.
C2DArray::GetAt¶
Get a text value from the specified x and y coordinates
CString& C2DArray::GetAt(
CString& nX,
CString& nY);
Parameters
CString& nX - x coordinate to get the text value from
CString& nY - y coordinate to get the text value from
Return Value
Returns the text value as CString&
C2DArray::GetAtLong¶
Get a numeric value from the specified x and y coordinates
const long C2DArray::GetAtLong(
CPoint& coordinates);
Parameters
CPoint& coordinates - x and y coordinates to get the numeric value from
Return Value
Returns the numeric value as a long integer
C2DArray::Load¶
Loads the specified 2da resource
void C2DArray::Load(
CResRef& res);
Parameters
CResRef& res - resource reference of the 2da file to load
Remarks
The disassembly below shows the following:
in a loop, loading of 17 strings and turning them into resource references with the
CResRef::CResRef
class methodpassing the newly created CResRef (resource reference) to the
C2DArray::Load
class method to load the specified .2da file resourcenote: esi is compared to 18 decimal (0x12) and continues the loop is below that value:
cmp esi,12
,jb baldur.604232
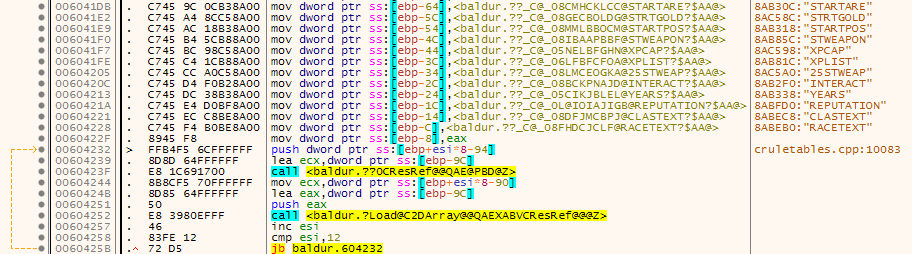
The equivalant in C++ might be:
string 2dalist[18] = {"LOADHINT", "MASTAREA", "NPCLEVEL", "TBPPARTY", "PDIALOG", "STARTARE", "STRTGOLD", "STARTPOS", "STWEAPON", "XPCAP", "XPLIST", "25STWEAP", "INTERACT", "YEARS", "REPUTATION" ,"CLASTEXT", "RACETEXT"};
CResRef ref;
C2DArray array;
for (int i = 0; i < 18; i++)
{
CResRef ref((const char*)2dalist[i]);
array.Load(ref);
}
C2DArray::LoadLines¶
Loads the specified 2da resource
void C2DArray::LoadLines(
CResRef& res);
Parameters
CResRef& res - resource reference of the 2da file to load